The selection sort algorithm sorts an array by repeatedly finding the minimum element (considering ascending order) from unsorted part and putting it at the beginning.
The algorithm maintains two subarrays in a given array.
1) The subarray which is already sorted.
2) Remaining subarray which is unsorted.
In every iteration of selection sort, the minimum element (considering ascending order) from the unsorted subarray is picked and moved to the sorted subarray.
Following example explains the above steps:
arr[] = 64 25 12 22 11
// Find the minimum element in arr[0...4]
// and place it at beginning
11 25 12 22 64
// Find the minimum element in arr[1...4]
// and place it at beginning of arr[1...4]
11 12 25 22 64
// Find the minimum element in arr[2...4]
// and place it at beginning of arr[2...4]
11 12 22 25 64
// Find the minimum element in arr[3...4]
// and place it at beginning of arr[3...4]
11 12 22 25 64
Programming code:
2) Remaining subarray which is unsorted.
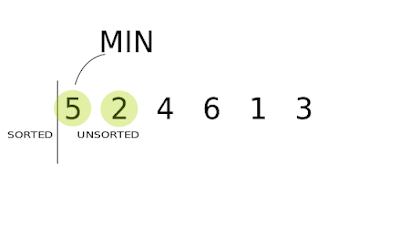
Following example explains the above steps:
arr[] = 64 25 12 22 11
// Find the minimum element in arr[0...4]
// and place it at beginning
11 25 12 22 64
// Find the minimum element in arr[1...4]
// and place it at beginning of arr[1...4]
11 12 25 22 64
// Find the minimum element in arr[2...4]
// and place it at beginning of arr[2...4]
11 12 22 25 64
// Find the minimum element in arr[3...4]
// and place it at beginning of arr[3...4]
11 12 22 25 64
Programming code:
import java.util.*;
class SelectionSort
{
void sort(int arr[])
{
int n = arr.length;
// One by one move boundary of unsorted subarray
for (int i = 0; i < n-1; i++)
{
// Find the minimum element in unsorted array
int min_idx = i;
for (int j = i+1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
// Swap the found minimum element with the first
// element
int temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
// Prints the array
void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i]+" ");
System.out.println();
}
// Driver code to test above
public static void main(String args[])
{
int no,i;
int arr[] =new int[10];
Scanner sc=new Scanner(System.in);
SelectionSort ob = new SelectionSort();
System.out.println("How many array elements want to create: ");
no=sc.nextInt();
System.out.println("Input "+no+" array elements: ");
for(i=0;i<no;i++)
arr[i]=sc.nextInt();
//print unsorted array
System.out.println("Unsorted array elements are: ");
for (i=0; i<no; ++i)
{
System.out.print(arr[i] + " ");
}
System.out.println();
ob.sort(arr);
System.out.println("Sorted array elements are: ");
ob.printArray(arr);
}
}
class SelectionSort
{
void sort(int arr[])
{
int n = arr.length;
// One by one move boundary of unsorted subarray
for (int i = 0; i < n-1; i++)
{
// Find the minimum element in unsorted array
int min_idx = i;
for (int j = i+1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
// Swap the found minimum element with the first
// element
int temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
// Prints the array
void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i]+" ");
System.out.println();
}
// Driver code to test above
public static void main(String args[])
{
int no,i;
int arr[] =new int[10];
Scanner sc=new Scanner(System.in);
SelectionSort ob = new SelectionSort();
System.out.println("How many array elements want to create: ");
no=sc.nextInt();
System.out.println("Input "+no+" array elements: ");
for(i=0;i<no;i++)
arr[i]=sc.nextInt();
//print unsorted array
System.out.println("Unsorted array elements are: ");
for (i=0; i<no; ++i)
{
System.out.print(arr[i] + " ");
}
System.out.println();
ob.sort(arr);
System.out.println("Sorted array elements are: ");
ob.printArray(arr);
}
}
Output:
How many array elements want to create:
5
How many array elements want to create:
5
Input 5 array elements:
4
3
5
7
1
4
3
5
7
1
Unsorted array elements are:
4 3 5 7 1
4 3 5 7 1
Sorted array elements are:
0 0 0 0 0 1 3 4 5 7
0 0 0 0 0 1 3 4 5 7